I'm interested whether different gpus give different results when copying from host to device memory, if some of you could compile and run the following program it would be handy!
gpuz:
Results over 3 runs (milliseconds):
#1 14.420
#2 14.080
#3 14.713
average: 14.404333
Code:
/*
* copyTest.cu
*
* Time a copy of how ever many megabytes to the device
*/
#include <stdio.h>
#define MB 32 //Number of megabytes to transfer
int main (void) {
int size = (MB*1024*1024) / sizeof(int); //number of cells needed for MB
int *a = (int*) malloc(size * sizeof *a);
int *dev_a;
printf("Copy Operation Test\n");
//Fill array with garbage
for (int i=0; i<size; i++) {
a[i] = i+i;
}
printf("Int size: %i bytes, size of array: %i MB\n", sizeof(int), MB);
cudaEvent_t start, stop;
float elapsedTime;
cudaEventCreate(&start);
cudaEventCreate(&stop);
//event start
cudaEventRecord(start,0);
//Allocate
cudaMalloc((void**) &dev_a, size * sizeof(int));
//Copy FROM, TO, NUMBER OF BYTES, DIRECTION
cudaMemcpy(dev_a, a, size * sizeof(int), cudaMemcpyHostToDevice);
//event stop
cudaEventRecord(stop, 0);
cudaEventSynchronize(stop);
cudaEventElapsedTime(&elapsedTime, start, stop);
printf("Time: %3.3f ms\n", elapsedTime);
//Tidy up
free(a);
cudaFree(dev_a);
cudaEventDestroy(start);
cudaEventDestroy(stop);
printf("Done!\n");
while( getchar() != '\n' );
return 0;
}
gpuz:
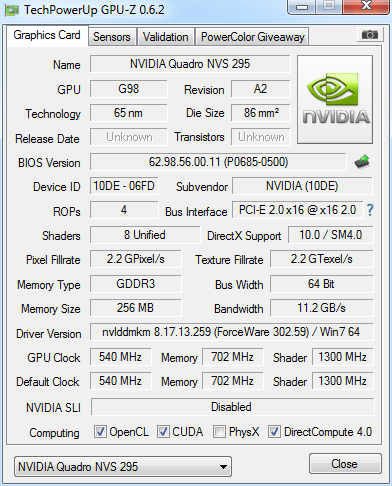
Results over 3 runs (milliseconds):
#1 14.420
#2 14.080
#3 14.713
average: 14.404333
Last edited: